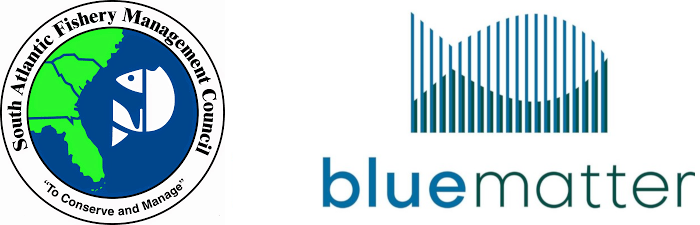
Creating Operating Models from BAM Output
Operating-Models.Rmd
Introduction
This article describes the process to import assessments conducted
with Beaufort Assessment Model (BAM) and create multi-fleet operating
models (OMs) in the openMSE
framework.
Prerequistes
The latest versions of MSEtool
and
bamExtras
need to be installed from GitHub:
install.packages('pak')
pak::pkg_install('nikolaifish/bamExtras')
pak::pkg_install('blue-matter/MSEtool')
These are the package versions used to create this article:
packageVersion('bamExtras')
#> [1] '0.0.1'
packageVersion('MSEtool')
#> [1] '3.7.9999'
OM Specifications
The number of simulations (nsim
) and number of
projection years (proyears
) need to be specified for an OM.
Here we are setting them as global variables so that each OM we create
has the same number of stochastic simulations and projection years:
nsim <- 50 # number of simulations
proyears <- 20 # number of projection years
An OM also requires the specification of an Observation Model. The Observation Model is used to simulate fishery data to be used be management procedures in the projection phase of the closed-loop simulation testing.
We are using Perfect_Info
as the fishery data are not
currently used within the management options (only static management
options are currently being explored). This can be changed later if
dynamic management options that respond to the observed fishery data are
added to the analysis.
Obs_Model <- Perfect_Info
Case Study Stocks
The current analysis is focused on the Red Snapper and the Gag Grouper stocks. The MSE framework is flexible, and more stocks can be added to the analysis.
Here we demonstrate creating OMs for the two case study stocks, and a third stock the Black Seabass.
The most recent BAM assessments are used:
Import SEDAR Assessments
The BAM assessments are imported from the bamExtras
package:
RS_rdat <- bamExtras::rdat_RedSnapper |> bamExtras::standardize_rdat()
GG_rdat <- bamExtras::rdat_GagGrouper |> bamExtras::standardize_rdat()
BS_rdat <- bamExtras::rdat_BlackSeaBass |> bamExtras::standardize_rdat()
Create OMs
The BAM2MOM
function is used to create the
openMSE
operating model objects:
RS_OM <- BAM2MOM(RS_rdat, stock_name='Red Snapper')
GG_OM <- BAM2MOM(GG_rdat, stock_name='Gag Grouper')
BS_OM <- BAM2MOM(BS_rdat, stock_name='Black Seabass')
These OMs can now be used within the openMSE
framework.
However, before doing so, it is important to understand the fleet
structure within each OM.
Define Fleet Structure
fleet_names_df <- dplyr::bind_rows(
data.frame(Stock='Red Snapper', Fleets=names(RS_OM@Fleets[[1]])),
data.frame(Stock='Gag Grouper', Fleets=names(GG_OM@Fleets[[1]])),
data.frame(Stock='Black Seabass', Fleets=names(BS_OM@Fleets[[1]]))
)
fleet_names_df |> dplyr::filter(Stock=='Red Snapper')
#> Stock Fleets
#> 1 Red Snapper cHL
#> 2 Red Snapper rHB
#> 3 Red Snapper rGN
#> 4 Red Snapper cHL.D
#> 5 Red Snapper rHB.D
#> 6 Red Snapper rGN.D
fleet_names_df |> dplyr::filter(Stock=='Gag Grouper')
#> Stock Fleets
#> 1 Gag Grouper cHL
#> 2 Gag Grouper cDV
#> 3 Gag Grouper rHB
#> 4 Gag Grouper rGN
#> 5 Gag Grouper cHL.D
#> 6 Gag Grouper rHB.D
#> 7 Gag Grouper rGN.D
fleet_names_df |> dplyr::filter(Stock=='Black Seabass')
#> Stock Fleets
#> 1 Black Seabass cHL
#> 2 Black Seabass cPT
#> 3 Black Seabass rHB
#> 4 Black Seabass rGN
#> 5 Black Seabass cGN.D
#> 6 Black Seabass rHB.D
#> 7 Black Seabass rGN.D
In order to combine these single stock OMs together, each OM must have the same fleet structure for all stocks in the OM, even if those fleets are not actively fishing on a particular stock.
The stocks have some fleets in common (e.g., Commercial hook and line (cHL)) and some unique fleets (e.g., Gag Grouper Commercial dive (cDV)). The landings and discards are also modeled as separate fleets in the BAM models (indicated by ā.Dā).
The landing and discard components for each fleet will be combined into a single fleet with a selectivity and retention curve.
The Black Seabass stock has two commercial fleets (cHL and cPT), but the discards are reported for two fleets combined (cGN.D). To deal with this, the cHL and cPT landing fleets will first be combined together, before the discards are added for the combined fleet.
fleet_df <- data.frame(Code=unique(fleet_names_df$Fleets))
fleet_df$Name <- c('Commercial Line',
'Recreational Headboat',
'General Recreational',
'Commercial Line - Discard',
'Recreational Headboat - Discard',
'General Recreational - Discard',
'Commercial Dive',
'Commercial Pot',
'Commercial General - Discard')
fleet_df$Mapping <- c(1,2,3,1,2,3,4,1,1)
fleet_df$Type <- 'Landing'
fleet_df$Type[grepl('\\.D', fleet_df$Code)] <- 'Discard'
fleet_df
#> Code Name Mapping Type
#> 1 cHL Commercial Line 1 Landing
#> 2 rHB Recreational Headboat 2 Landing
#> 3 rGN General Recreational 3 Landing
#> 4 cHL.D Commercial Line - Discard 1 Discard
#> 5 rHB.D Recreational Headboat - Discard 2 Discard
#> 6 rGN.D General Recreational - Discard 3 Discard
#> 7 cDV Commercial Dive 4 Landing
#> 8 cPT Commercial Pot 1 Landing
#> 9 cGN.D Commercial General - Discard 1 Discard
The Mapping
variable describes how the fleets will be
combined together. The end result will be a fleet structure with four
fleets for each stock:
- Commercial Line
- Recreational Headboat
- General Recreational
- Commercial Dive
Define Discard Mortality
The discard mortality needs to be added to the OMs so that the historical effort can be adjusted to account for the fish that were caught and released alive, and so that the discard mortality can be modified in the projections.
The discard mortality for Red Snapper is reported in Table 6 of SEDAR
73. TheYear
variable indicates the first year the
discard mortality changes:
discard_mortality_RS <- dplyr::bind_rows(
data.frame(Stock='Red Snapper',
Code='cHL',
Year=c(1900, 2007, 2017),
Prob_Dead=c(0.48, 0.38, 0.36)),
data.frame(Stock='Red Snapper',
Code='rHB',
Year=c(1900, 2011, 2018),
Prob_Dead=c(0.37, 0.26, 0.25)),
data.frame(Stock='Red Snapper',
Code='rGN',
Year=c(1900, 2011, 2018),
Prob_Dead=c(0.37, 0.28, 0.26))
)
The discard mortality for Gag Grouper is reported in SEDAR 71, with a fixed discard mortality of 0.4 for the commerical fleet, and 0.25 for the recreational fleets for all years:
discard_mortality_GG <- dplyr::bind_rows(
data.frame(Stock='Gag Grouper',
Code='cHL',
Year=1900,
Prob_Dead=0.4),
data.frame(Stock='Gag Grouper',
Code='rHB',
Year=1900,
Prob_Dead=0.25),
data.frame(Stock='Gag Grouper',
Code='rGN',
Year=1900,
Prob_Dead=0.25)
)
The discard mortality for Black Seabass is reported in Section 2.2.1 in SEDAR 76. Here the average discard mortality is calculated for the combined commercial line and pot fleets:
discard_mortality_BS <- dplyr::bind_rows(
data.frame(Stock='Black Sea Bass',
Code='cHL',
Year=c(1900, 2007),
Prob_Dead=c(mean(0.14,0.19), mean(0.14, 0.068))
),
data.frame(Stock='Black Sea Bass',
Code='rHB',
Year=1900,
Prob_Dead=0.152),
data.frame(Stock='Black Sea Bass',
Code='rGN',
Year=1900,
Prob_Dead=0.137)
)
The stock-specific discard mortality dataframes are combined together:
discard_mortality <- dplyr::bind_rows(discard_mortality_RS,
discard_mortality_GG,
discard_mortality_BS)
Aggregate Fleets
Next, the Aggregate_Fleets
function is used to aggregate
the fleets together according to the mapping defined in
fleet_df
and the discard mortality are added from
discard_mortality
.
The Aggregate_Fleets
function does five things:
- Combine any landing fleets that are mapped together in
fleet_df
(here only applies to Black Seabass) - Add the discard mortality to each fleet
- Combine the discard fleets with the landing fleets to produce a single fleet with selectivity and retention curves
- Add dummy fleets (F=0 for all years) for OMs that are missing some fleets
- Order the fleets for all OMs
RS_OM <- Aggregate_Fleets(RS_OM, fleet_df, discard_mortality)
GG_OM <- Aggregate_Fleets(GG_OM, fleet_df, discard_mortality)
BS_OM <- Aggregate_Fleets(BS_OM, fleet_df, discard_mortality)